
C 数组
学习C - C数组
什么是数组?
数组包含固定数量的具有相同类型的数据项。
数组中的数据项被称为元素。
我们在名字之后放置一个方括号 []
之间的数字。
long numbers[10];
方括号之间的数字定义了数组包含的元素数量。
它被称为数组维。
数组索引值从零开始,而不是一个。
以下代码显示如何平均存储在数组中的十个等级。
#include <stdio.h>
int main(void)
{
int grades[10]; // Array storing 10 values
unsigned int count = 10; // Number of values to be read
long sum = 0L; // Sum of the numbers
float average = 0.0f; // Average of the numbers
printf("\nEnter the 10 grades:\n"); // Prompt for the input
// Read the ten numbers to be averaged
for(unsigned int i = 0 ; i < count ; ++i)
{
printf("%2u> ",i + 1);
scanf("%d", &grades[i]); // Read a grade
sum += grades[i]; // Add it to sum
}
average = (float)sum/count; // Calculate the average
printf("\nAverage of the ten grades entered is: %.2f\n", average);
return 0;
}
上面的代码生成以下结果。
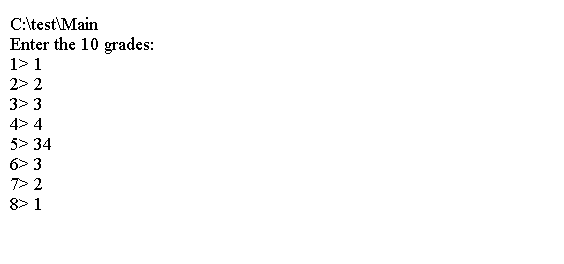
我们可以使用[]语法定义数组。
例如,我们定义int和char的数组。
int number [5];char chars [10];
我们也可以从struct构造数组。
struct employee{ int id; char name[10]; char country[5]; }; struct employee list[5];
例子
以下代码显示数组中的所有值。
您可以通过索引访问数组元素值。
#include <stdio.h>
int main(void)
{
int grades[10];
unsigned int count = 10; // Number of values to be read
long sum = 0L; // Sum of the numbers
float average = 0.0f; // Average of the numbers
printf("\nEnter the 10 grades:\n"); // Prompt for the input
// Read the ten numbers to be averaged
for(unsigned int i = 0 ; i < count ; ++i)
{
printf("%2u> ",i + 1);
scanf("%d", &grades[i]); // Read a grade
sum += grades[i]; // Add it to sum
}
average = (float)sum/count; // Calculate the average
for(unsigned int i = 0 ; i < count ; ++i) {
printf("\nGrade Number %2u is %3d", i + 1, grades[i]);
}
printf("\nAverage of the ten grades entered is: %.2f\n", average);
return 0;
}
上面的代码生成以下结果。
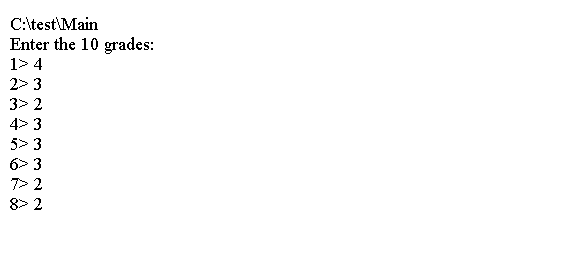
例2
声明一个数组后,我们可以设置并获取数组。
#include <stdio.h>
struct employee{
int id;
char name[10];
char country[5];
};
int main() {
// define array
int numbers[5];
char chars[10];
struct employee list[5];
// insert data
int i;
for(i=0;i<5;i++){
numbers[i] = i;
list[i].id = i;
sprintf(list[i].name,"usr %d",i);
sprintf(list[i].country,"DE");
}
sprintf(chars,"hello c");
// display data
for(i=0;i<5;i++){
printf("%d %c\n",numbers[i],chars[i]);
printf("struct. id: %d, name: %s, country : %s \n",
list[i].id,list[i].name,list[i].country);
}
printf("%s\n",chars);
return 0;
}
上面的代码生成以下结果。
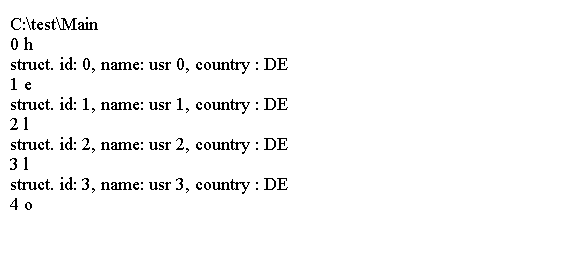
运算符地址
运算符的地址&,返回其操作数的存储器地址。
以下代码显示如何输出一些变量的地址:
#include<stdio.h>
int main(void)
{
// Define some integer variables
long a = 1L;
long b = 2L;
long c = 3L;
// Define some floating-point variables
double d = 4.0;
double e = 5.0;
double f = 6.0;
printf("A variable of type long occupies %u bytes.", sizeof(long));
printf("\nHere are the addresses of some variables of type long:");
printf("\nThe address of a is: %p The address of b is: %p", &a, &b);
printf("\nThe address of c is: %p", &c);
printf("\n\nA variable of type double occupies %u bytes.", sizeof(double));
printf("\nHere are the addresses of some variables of type double:");
printf("\nThe address of d is: %p The address of e is: %p", &d, &e);
printf("\nThe address of f is: %p\n", &f);
return 0;
}
上面的代码生成以下结果。
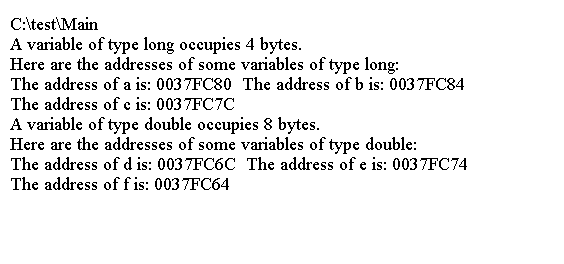
数组和地址
这是一个包含四个元素的数组的声明:
long number[4];
数组名称 number
用于标识数组的内存地址。
索引值表示数组元素内存地址的偏移量。
以下代码为数组中的每个元素设置一个值,并输出每个元素的地址和内容:
#include <stdio.h>
int main(void)
{
int data[5];
for(unsigned int i = 0 ; i < 5 ; ++i)
{
data[i] = 12*(i + 1);
printf("data[%d] Address: %p Contents: %d\n", i, &data[i], data[i]);
}
return 0;
}
上面的代码生成以下结果。
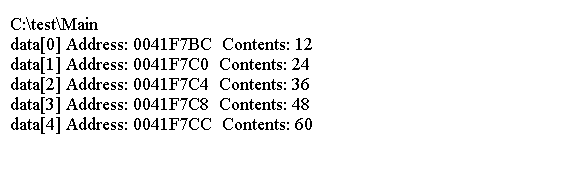
初始化数组
要初始化数组的元素,请在括号中指定初始值,并在声明中用逗号分隔。
例如:
double values[5] = { 1.5, 2.5, 3.5, 4.5, 5.5 };
如果初始值比元素少,则不初始化值的元素将被设置为0.因此,如果写入:
double values[5] = { 1.5, 2.5, 3.5 };
前三个元素将用大括号之间的值初始化,最后两个元素将被初始化为0。
要将整个数组初始化为零,请提供一个值为0的元素:
double values[5] = {0.0};
然后将使用0.0初始化整个数组。
当您指定初始值列表时,可以省略数组的大小。
编译器将假定元素的数量是列表中的值的数量:
int primes[] = { 2, 3, 5, 7, 11, 13, 17, 19, 23, 29};
数组的大小由列表中初始值的数量决定。
查找数组的大小
sizeof
运算符计算变量占用的字节数。
您可以将 sizeof
运算符应用于类型名称,如下所示:
printf("The size of a variable of type long is %zu bytes.\n", sizeof(long));
sizeof运算符也使用数组。
假设您使用以下语句声明一个数组:
double values[5] = { 1.5, 2.5, 3.5, 4.5, 5.5 };
您可以使用以下语句输出数组占用的字节数:
printf("The size of the array, values, is %zu bytes.\n", sizeof values);
这将产生以下输出:
The size of the array, values, is 40 bytes.
因此,您可以使用 sizeof
运算符来计算数组中的元素数量:
size_t element_count = sizeof values/sizeof values[0];
element_count
的类型为 size_t
,因为sizeof运算符产生size_t类型值。
可以将sizeof运算符应用于数据类型,以下代码返回双维数组的数组维度。
size_t element_count = sizeof values/sizeof(double);
用于计算数组长度或维度的完整源代码如下。
#include <stdio.h>
int main(void)
{
double values[5] = { 1.5, 2.5, 3.5, 4.5, 5.5 };
size_t element_count = sizeof(values)/sizeof(values[0]);
printf("The size of the array is %zu bytes ", sizeof(values));
printf("and there are %u elements of %zu bytes each\n", element_count, sizeof(values[0]));
return 0;
}
上面的代码生成以下结果。

例3
当您使用循环来处理数组中的所有元素时,可以使用sizeof运算符。
例如:
#include <stdio.h>
int main(void)
{
double values[5] = { 1.5, 2.5, 3.5, 4.5, 5.5 };
double sum = 0.0;
for(unsigned int i = 0 ; i < sizeof(values)/sizeof(values[0]) ; ++i){
sum += values[i];
}
printf("The sum of the values is %.2f", sum);
return 0;
}
上面的代码生成以下结果。
