
C While
学习C - C While
while循环重复指定逻辑表达式的一组语句。
如果表达式的计算结果为true,则while循环将继续。
while循环的一般语法如下:
while( expression ) statement1; statement2;
在开始时测试持续while循环的条件。
如果表达式开始为false,则不会执行循环语句。
如果循环条件开始为真,则循环体必须将其更改为false以结束循环。
以下代码显示了如何使用while循环来求和整数
#include <stdio.h>
int main(void)
{
unsigned long sum = 0UL; // The sum
unsigned int i = 1; // Indexes through the integers
unsigned int count = 0; // The count of integers to be summed
printf("\nEnter the number of integers you want to sum: ");
scanf(" %u", &count);
// Sum the integers from 1 to count
while(i <= count)
sum += i++;
printf("Total of the first %u numbers is %lu\n", count, sum);
return 0;
}
上面的代码生成以下结果。

例子
以下代码显示了如何在语法时使用。
#include <stdio.h>
int main() {
int num = 0;
while(num<10){
printf("data %d\n",num);
num++;
}
return 0;
}
上面的代码生成以下结果。
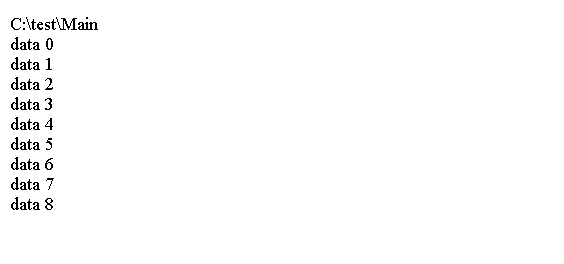
注意
在这个例子中,你将在for循环中嵌套一个while循环。
#include <stdio.h>
int main(void)
{
unsigned long sum = 1UL; // Stores the sum of integers
unsigned int j = 1U; // Inner loop control variable
unsigned int count = 5; // Number of sums to be calculated
for(unsigned int i = 1 ; i <= count ; ++i)
{
sum = 1UL; // Initialize sum for the inner loop
j=1; // Initialize integer to be added
printf("\n1");
// Calculate sum of integers from 1 to i
while(j < i)
{
sum += ++j;
printf(" + %u", j); // Output +j - on the same line
}
printf(" = %lu", sum); // Output = sum
}
printf("\n");
return 0;
}
上面的代码生成以下结果。
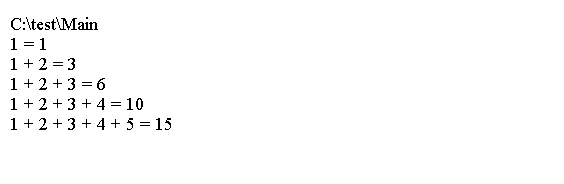
do-while循环
do-while循环测试循环是否应该继续,循环语句或语句块总是至少执行一次。
do-while循环的一般表示是:
do { /* Statements for the loop body */ } while(expression);
如果循环体只是一个语句,则可以省略大括号。
在do-while循环中的括号后面有一个分号。
在do-while循环中,如果expression的值为true(非零),则循环继续。
只有当表达式的值变为false(零)时,循环才会退出。
以下代码显示了如何使用do-while循环来反转正数的数字:
#include <stdio.h>
int main(void)
{
unsigned int number = 123; // The number to be reversed
unsigned int reversedNumber = 0;
unsigned int temp = 0; // Working storage
temp = number; // Copy to working storage
// Reverse the number stored in temp
do
{
// Add rightmost digit of temp to reversedNumber
reversedNumber = 10*reversedNumber + temp % 10;
temp = temp/10; // and remove it from temp
} while(temp); // Continue as long as temp is not 0
printf("\nThe number %u reversed is %u reversedNumber ehT\n", number, reversedNumber );
return 0;
}
任何非零整数将转换为true。布尔值false对应于零。
上面的代码生成以下结果。
